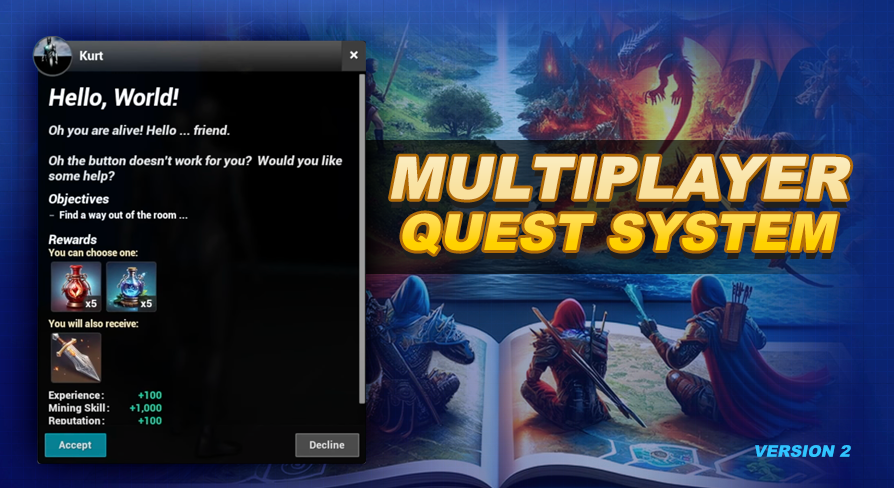
User Interfaces
UE5 Quest System Version: 2.0Location of the UIs
All the user interfaces for the project are located in the UIs/ folder. Inside this folder, each subfolder corresponds to a different unique interface. For the main HUD UI_QuestSystem_HUD, you can find it directly in this base UIs/ folder.
Quest HUD (UI_QuestSystem_HUD)
This is the main HUD, the central element for the quest system, handling all tasks related to the user interface. While we can trigger certain actions, like displaying quest windows or simple alerts, through the AC_QuestSystem_PlayerController component, the actual functionality for these actions is located within this HUD. If you need a reference to the player's quest HUD you can get this from the AC_QuestSystem_PlayerController, the variable named questHUD is the one you are looking for.
Moving around the placement of things shown on the HUD
To adjust the positions of elements like the Quest Tracker, Quest Window, or alerts on the HUD, navigate to the Designer tab of the Quest HUD. In this tab, use the Hierarchy panel to select the anchors associated with these elements. Once selected, you can move them to your preferred location on the screen.
Controlling the Mouse Cursor and Input Mode
By default the system will attempt to manage the mouse cursor and input when quest related windows are opened and closed. If you would like to disable the system from handling this, such as for top down games where you always want to show the mouse you can set the controlInputAndMouse? boolean to false in the UI_QuestSystem_HUD.
Quest Type Coloring
The coloring related to the quest type (title and border) is set inside the getQuestColor function found inside the Quest HUD.
Quest Interaction Notice
The Quest HUD also manages the visibility of the Interact notice you see when you get in range of an interactable, but this is driven by event dispatchers. The event dispatchers are part of the AC_QuestSystem_PlayerController. This is where the core functionality of the interaction system is actually handled. If you need more information or guidance on how this works, you should refer to the Interaction System page.
Quest Input Routing
Input routing to the quest window and quest log is also handled within the quest HUD. This is for input bindings that are used when a mouse/pointer is not, like a gamepad. View the Input & Key Bindings chapter to learn more about how input works.
Quest Sounds
The HUD is also the central point for all UI related sound fx you hear in the quest system. There's a variable called questSounds map, which defines the sound associated with each action within the system. When a specific sound needs to be played, the playQuestSound event in this blueprint is called. This event uses the questSounds map to determine which sound effect to play for a given action.
All of the sounds that you hear in the default setup are from the Engine provided content, using pitch and volume modifiers on the fly. Since everything comes through this one central point you can simply disconnect the pitch and volume modifiers if and when you need to switch the sounds to play cues instead. I advise not deleting the inputs on this event or you will get errors in other blueprints where random floats (to modulate on the fly) are being used to power the pitch values when this event is called. You can also use the "Find References" right click option from the list on the playQuestSound event to see where it is being called in the system if you would like to remove those extra bits.
Helpful Functions found in the Quest HUD
Included you will find a number of helpful functions for some of the most common needs:
Quest Window (UI_QuestWindow)
The quest window is the interface the player engages with when accepting or completing a quest. It is also used with our Turn In Item quest objective.
Quest Text (UI_QuestWindow_Text)
The Quest Text is a crucial element in both the quest window and the quest log, providing details about the quest. If you're aiming to alter the appearance or position of the text within these parts of the interfaces, the Quest Text blueprint is most likely the component you'll need to adjust.
Scene Capture Component
The scene capture component can be configured on your actor using the BPI_Quest_Actor_Options's getCaptureTransformOptions.
Distance Closer
The distance checker will close a quest window if it is initiated from an actor. This distance check is handled inside the UI_QuestSystem_HUD through the function onDistanceCheck (find references on this function to see where it is called in the controller component). You can disable this functionality by setting CloseQuestWindowOnMaxDistance? to false in the UI_QuestSystem_HUD. This function is called when you leave the range of the interact actor (from the controller component). If you want to change the max distance this can be done using the Quest Actor Options BPI.
Quest Tracker (UI_QuestTracker)
The Quest Tracker on the HUD displays the player's active quests. When a player accepts a new quest, it's automatically listed in the Quest Tracker. If players wish to stop tracking a specific quest, they can do so by selecting the quest in the quest log and clicking the 'untrack' button. Once a quest is untracked, a 'Track' button will appear. Clicking this 'Track' button will then re-enable the quest's tracking on the HUD, making its details visible again in the Quest Tracker.
Quest Log (UI_QuestLog)
The quest log is a feature where players can view all their ongoing quests at any time. To open or close the quest log, players use the enhanced input action called IA_Quest_Log. By default, the 'L' key on the keyboard and the Right Shoulder button on a gamepad are set to toggle the quest log. If you want to change these keybindings, you can do so in the IMC_QuestSystem located in the Blueprints/Input folder. For a deeper understanding of how inputs and keybindings are configured in this project, refer to the Input & Keybindings chapter.
Abandoning Quests
Players also have the option to abandon quests from the quest log that are currently in progress. This can be done by clicking the abandon button at the bottom left of the quest log after selecting a quest that is in progress. When a quest is abandoned, it is removed from the players log, and tracking, and its state is set back to Not Available. By default if the quest is ready to be turned in the quest can not be abandoned, this is to avoid accidental drops by the player.
To prevent players from abandoning a specific quest, you can set the Can Abandon Quest? boolean to false in the quest options section in the data table for the quest.
When a quest is abandoned, the system is designed to execute a sequence of quest events. By default, the system triggers the events that are set up in the "Failed" quest state. However, you have the flexibility to change this so that it triggers a different set of events tied to another quest state. This can be configured using the onAbandonRunEvents option in the Quest Options.
One thing you will want to keep in mind is that the Run On Reload? boolean has no effect and will not rerun on the next game session when events are initiated through the abandon quest handler.
Another thing you will want to keep in mind is if your quest is designed to be auto-accepted by players, it's wise to disable the abandon feature for such quests. This prevents a scenario where the player tries to abandon the quest, only to have it reappear and auto-accept again, which can be frustrating for the player.
Sharing Quests
The ability to share quests also occurs from the quest log, however this button will only appear in multiplayer games, and is only accessible if the CanShareQuest? Quest option is set to true (it is by default).
In addition to sharing quests you can also share quest objective progress, this is the CanShareProgress? boolean inside the Quest Objective Options, for each of your objectives.
Grouping Quests
Grouping of quests occurs by defining a Quest Log Group in the data table for your quest. All quests with matching Quest Log Groups will be stacked together when shown in the quest log. Any quest not associated with a group will be placed in the Other Quests group. This label is set inside the refreshQuestLog function in the UI_QuestLog.
Hiding the Completed Quests Group in the Quest Log
There is a boolean variable called showCompletedQuests? inside the UI_QuestLog that can be set to false to hide the Completed Quests group that is shown by default in the quest log. Completed quests will have the word "(Completed)" appended to the end of the quest title in the log. If you would like to remove this text this is being added inside the renderButton function of the UI_QuestLog_Quest.
Sorting Quests within Groups
There are no built in methods to sort quests within groups, by default the data will be unsorted. If you wanted to sort the groups you would do so in the rebuildGroup function of the UI_QuestLog_Group. Look for the gold comment in this function. This is where I recommend adding something like this.
Sorting Quest Groups
There are no built in sorting methods with the quest groups but if you wanted to make this change you would do so in the refreshQuestLog function of the UI_QuestLog. Look for the gold comment in this function. This is where I recommend adding something like this.
Quest Alerts
In the quest system, there are two sizes of quest alerts to communicate with players: small and large.
Small Alert (UI_QuestAlert_Small): This type is for indicating progress or delivering quest-progress related messages, such as announcing the current objective count progress. When a small alert is spawned via the showSmallAlert function on the UI_QuestSystem_HUD, it attaches to the anchorQuestAlertsSmall overlay within the UI_QuestSystem_HUD. These alerts can be customized in terms of text and color, and you can also set a duration to define how long the alert should remain visible unless it's replaced by a new one. There is also a quest event (Simple Alert) that can be used to spawn the small alerts during quest progression.
Large Alert (UI_QuestAlert_Large): Used for highlighting significant updates, like changes in the quest state. Large alerts are spawned by the showLargeAlert function within the UI_QuestSystem_HUD, it attaches to the anchorQuestAlertsLarge overlay. Similar to small alerts, you can customize the text and color for large alerts. Additionally, there's an option to remove any existing alerts when a new one is displayed.
These features allow for dynamic communication with players, ensuring they're kept informed about their quest progress and any important developments.
Quest Interact Notice (UI_Quest_Interact)
The quest interact notice is integrated within the UI_QuestSystem_HUD, and its visibility is managed by two functions: showInteractNotice and hideInteractNotice, both of which are located in the UI_QuestSystem_HUD. The activation of these functions is governed by event dispatchers found in the AC_QuestSystem_PlayerController.
The keybinding displayed in this interact notice widget is sourced from the IMC_QuestSystem located in the Blueprints/Input/ folder. By default, the widget uses the first keybinding listed in the input mapping context for mouse/keyboard interactions. For gamepad users, the widget displays the keybinding listed at index 1 in the input mappings. The system determines whether to show the mouse/keyboard or gamepad keybinding based on the detectGamepad function, which evaluates the input type during each interaction.
Quest Timer UI (UI_QuestTimer)
The Quest Timer UI widget appears when a Quest Timer Quest Event is triggered, using the specified ui key in the EventData. This widget serves as a visual indicator of the countdown happening on the server. The actions that occur upon the timer's expiration are managed within the Quest Timer Quest Event itself, not in the UI.
The title of a quest timer is set by the value you define for the ui key set in the EventData for the Quest Timer. If you leave the value of ui blank it will default to the quest's title.
When the quest timer reaches zero, the UI will display "!!!!!". This is set into the timer mechanism initiated from the Event Construct of the UI if you would like to change it.
Additionally, the widget includes a visual cue: it starts flashing red when the timer falls below 10 seconds. This is an animation designed to alert players that time is running out. You have the option to adjust this 10-second threshold within the timer event found on the construct.
Demo World UI Quest
In the demo world there is a bubble that if you step in will present a UI where an entire quest can be completed within it. This blueprint is not stored with the others since it is specific to the demo world and not the quest system. You can find this blueprint in the Demo/UI/ folder.